Getting Started with Golang for DevOps: Best Practices and Tools
Golang, also known as Go, is a programming language developed by Google that is gaining popularity among DevOps engineers. Its simplicity, performance, and support for concurrent programming make it an excellent choice for building backend services and command-line tools. This blog post will explore how to get started with Golang for DevOps and some best practices and tools to use when building applications.
Why Golang for DevOps?
Golang is an excellent choice for DevOps engineers for several reasons:
- Performance: Golang is known for its performance and is often used to build high-performance systems. It has a built-in garbage collector and supports concurrent programming, which makes it ideal for building scalable systems.
- Simplicity: Golang's syntax is simple and easy to understand, making it easy to write, maintain and troubleshoot the code.
- Cross-platform: Golang is a cross-platform language. It can be compiled for different platforms and architectures, which makes it easy to deploy the same codebase to different environments.
- Great community: Golang has a large and active community of developers who contribute to the language, libraries and tools.
Getting Started with Golang
Before building applications with Golang, we need to set up our development environment.
Installing Golang
The first step is to install Golang on your machine. You can download the latest version of Golang from the official website: https://golang.org/dl/.
Once you have downloaded and installed Golang, you should add its bin directory to your system's PATH variable so that you can run Go commands from the command line.
Setting up a workspace
Golang uses a concept called a "workspace", a directory containing all of the source code and dependencies for a project. The workspace has the following structure:
workspace/
- cmd/
- internal/
- pkg/
The cmd
directory contains the entry point to your application, and the internal
directory should have source code not meant to be accessed by anything external to the project. Finally, the pkg
should contain the project's source code which is also accessible externally.
Hello World
Now that we have set up our development environment let's write our first Go program. Create a new file named main.go
in the cmd
directory of your workspace and add the following code:
package main
import "fmt"
func main() {
fmt.Println("Hello, World!")
}
This program is a simple "Hello World" application that uses the fmt
package to print the string "Hello, World!" to the console.
To run the program, open a terminal and navigate to your workspace's cmd
directory. Then, run the following command:
go run main.go
You should see the output "Hello, World!" in the terminal.
Best Practices
Now that we have a basic understanding of how to write Go programs let's explore some best practices for building applications with Golang.
Code organization
Golang follows the concept of "convention over configuration". This means that the Go community has agreed on some conventions for organizing code, which makes it easy to understand and maintain the codebase.
One of the most essential conventions is the use of the package system. Each Go file should belong to a package, and the package name should be the same as the name of the directory it's in. For example, if you have a package named example
in a directory called example
, all the Go files in that directory should have the line package example at the top.
Another important convention is the main
package and main
function. The main
package is the program's entry point, and the main
function is where the execution of the program starts.
Go Modules
Go Modules is the package management system for Go. It allows you to manage dependencies for your project and ensure that all builds are consistent across different environments.
To use Go Modules, you need to add a go.mod
file to the root of your project. This file contains the dependencies for your project and can be used to import and use packages from other Go projects.
Error Handling
Error handling is an essential aspect of programming, and Go provides a built-in mechanism for handling errors. The error
type is a built-in interface in Go and is used to represent an error.
A function should be checked and handled correctly when it returns an error. The idiomatic way to check for errors in Go is to use the if err != nil
pattern.
_, err := myFunc()
if err != nil {
// handle the error
}
Concurrency
Concurrency is a key feature of Go, allowing you to run multiple tasks in parallel. Go uses goroutines and channels to implement concurrency.
A goroutine is a lightweight thread of execution, and it is created using the keyword go
. A channel is a mechanism for communication between goroutines.
ch := make(chan int)
go func() {
ch <- 1
}()
fmt.Println(<-ch)
In this example, we create a channel and start a goroutine that sends the value 1 to the channel. Then, we receive the value from the channel and print it to the console.
Tools
Here are some popular tools and libraries that can be used when building applications with Golang:
- Gorilla Web Toolkit: A web toolkit that provides a set of libraries for building web applications.
- Gin: A web framework optimized for high performance and low overhead.
- Echo: A web framework similar to Gin but with more features.
- GoReplay: A tool for replaying and analyzing network traffic.
- Prometheus: A monitoring and alerting system for cloud-native applications.
Conclusion
Golang is an excellent choice for DevOps engineers due to its performance, simplicity, and support for concurrent programming. By following the best practices and using the right tools, you can build high-performance and scalable applications with Golang.
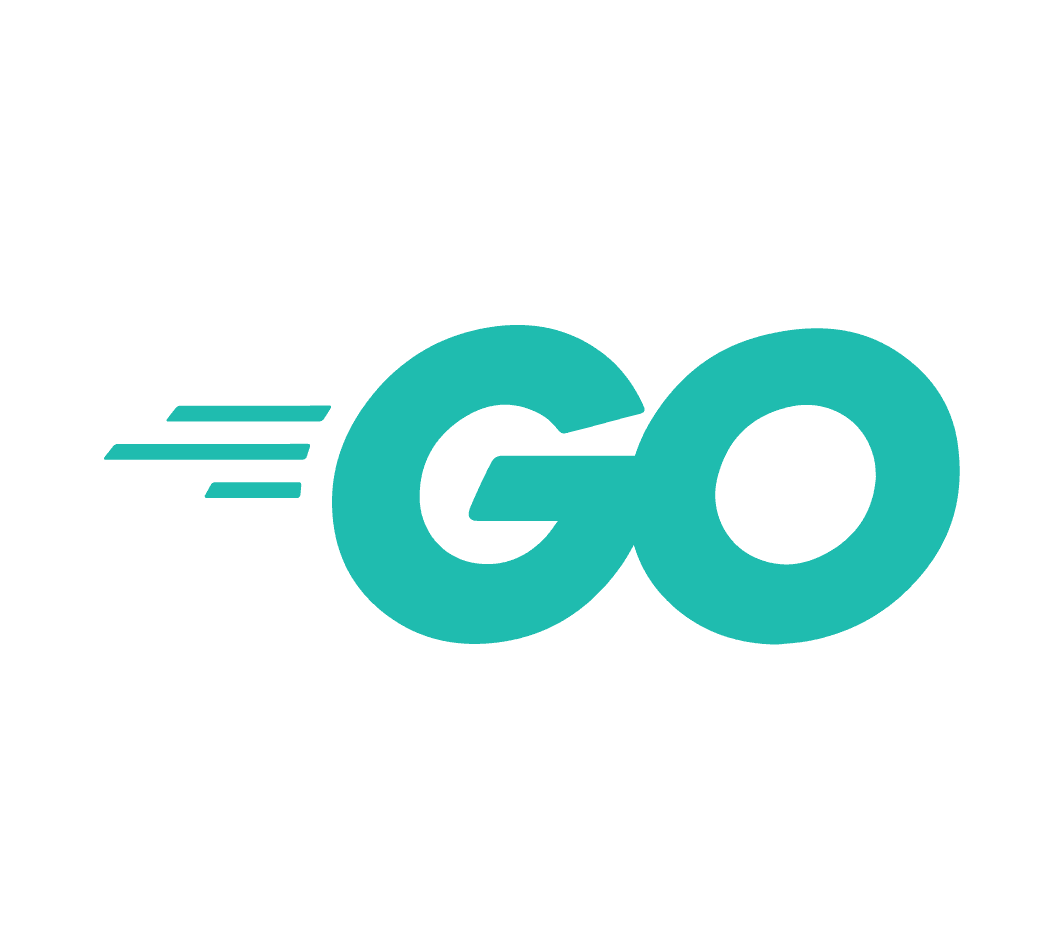
This post has covered the basics of getting started with Golang and some best practices and tools to use when building applications. Keep in mind that the Go community is constantly evolving and updating, so stay up-to-date with the latest developments and trends.